Introduction to Asynchronous Programming with C#
Asynchronous programming aims to maximize the use of resources, such as CPU time and memory, and to minimize the time it takes for a program to complete a task.
In today’s fast-paced digital world, users expect applications to be quick and responsive, even when dealing large amounts of data. To meet these demands, developers must turn to asynchronous programming. By breaking down long-running tasks into smaller, asynchronous tasks, we can ensure that our applications remain quick and responsive, even when processing large amounts of data.
Asynchronous programming allows the program to continue executing other tasks. At the same time, it waits for a task to complete, reducing the time it takes for a program to complete a task.
This article will explore how to implement asynchronous programming in C# using the Task class and the async keyword. We’ll also look at how to use the await keyword to wait for asynchronous tasks to complete and how to use the Task.WhenAll method to wait for multiple tasks to complete.
By the way, if you are interested in learning more about APIs, we also have several articles on this topic! Make sure to check them out! Like this one, about the GET method and How to Call GET API in C#!
So whether you’re a beginner or an experienced developer, this article will give you a solid understanding of asynchronous programming in C# and help you create fast and responsive applications.
Create a new C# Console App
To begin, we’ll need to create a new C# console application in Visual Studio.
- Open Visual Studio and click on “Create a new project.”
- Select “Console App (.NET 6)” from the list of templates.
- Give your project a name, for example “AsyncInCsharp” and click on “Create.”
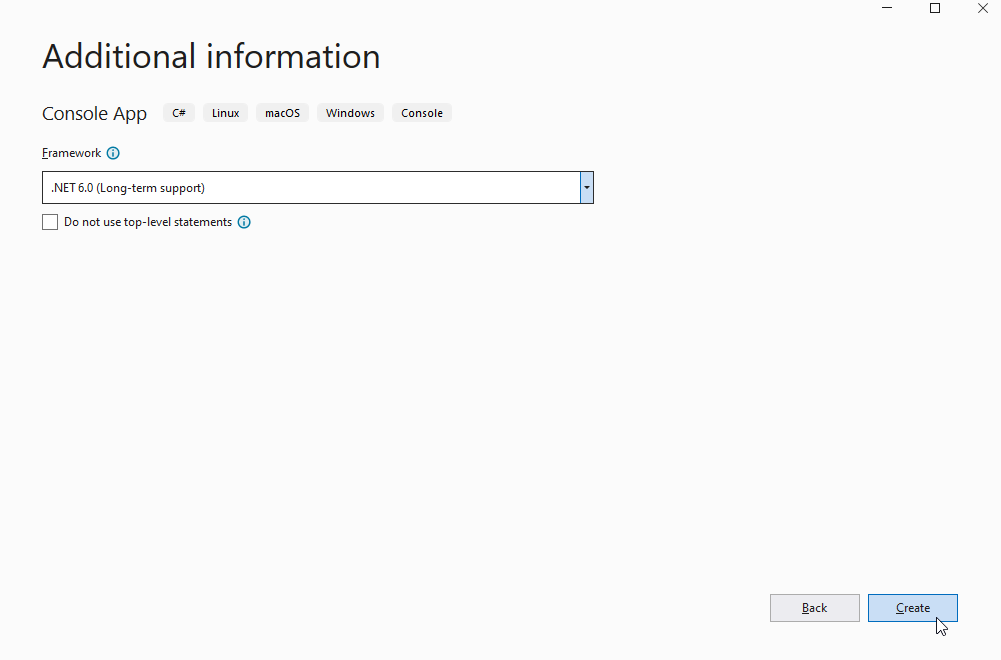
Getting Started with the Main Method
Let’s start with the Main method. The Main method is the entry point of our program and where all the magic happens. The first thing we need to do is add the async keyword to the Main method signature. This keyword tells C# that the Main method is asynchronous, meaning it can run multiple tasks simultaneously.
static async Task Main(string[] args) { }
Getting Data Asynchronously
The next step is to retrieve data from two different sources. In this case, we’re retrieving weather data and news articles. We do this by calling two separate methods, GetWeatherAsync and GetNewsAsync. These methods are asynchronous and retrieve data from the API. We use the HttpClient class to retrieve the data, which is part of the System.Net.Http namespace. HttpClient is a modern HTTP client that supports many advanced features, including asynchronous programming.
static async Task<string> GetWeatherAsync() { // Use a real weather API here var client = new HttpClient(); var weatherTask = client.GetStringAsync("https://api.openweathermap.org/data/2.5/weather?q=London,uk&appid=YOURAPIKEY"); var weatherResult = await weatherTask; return "Weather Data: " + weatherResult; } static async Task<string> GetNewsAsync() { // Use a real news API here var client = new HttpClient(); var newsTask = client.GetStringAsync("https://newsapi.org/v2/top-headlines?country=us&apiKey=YOURAPIKEY"); var newsResult = await newsTask; return "News Articles: " + newsResult; }
By the way, to make this work, you must have an API key from both openweathermap.org/ as well as newsapi.org/. By signing up you should be able to get an API key for both so don´t worry!
Calling both tasks in the Main Method
Now that both methods are implemented, we will have to call them both in the Main method. This can be done as usual as if it was a normal method. Like this:
static async Task Main(string[] args) { // Retrieve weather data asynchronously var weatherTask = GetWeatherAsync(); // Retrieve news articles asynchronously var newsTask = GetNewsAsync(); }
Waiting for Both Tasks to Complete
Once both methods have been called, we use the Task.WhenAll method to wait for both tasks to be complete. This method takes an array of tasks and blocks the calling thread until all tasks are completed.
static async Task Main(string[] args) { // Retrieve weather data asynchronously var weatherTask = GetWeatherAsync(); // Retrieve news articles asynchronously var newsTask = GetNewsAsync(); // Wait for both tasks to complete await Task.WhenAll(weatherTask, newsTask); }
Displaying the results
Once both the weather and news tasks are completed, we can then display the results. This is done using the Console.WriteLine method. The results of the tasks are stored in the Result property of the tasks, which is accessed like this:
static async Task Main(string[] args) { // Retrieve weather data asynchronously var weatherTask = GetWeatherAsync(); // Retrieve news articles asynchronously var newsTask = GetNewsAsync(); // Wait for both tasks to complete await Task.WhenAll(weatherTask, newsTask); // Display the results Console.WriteLine("Weather:"); Console.WriteLine(weatherTask.Result); Console.WriteLine(); Console.WriteLine("News:"); Console.WriteLine(newsTask.Result); }
In this code, we first print the word “Weather:” to the console and then the result of the weather task. Next, we print a blank line using Console.WriteLine() to create some space and then print the word “News:” followed by the result of the news task. Finally, we add a prompt for the user to exit the program:
static async Task Main(string[] args) { // Retrieve weather data asynchronously var weatherTask = GetWeatherAsync(); // Retrieve news articles asynchronously var newsTask = GetNewsAsync(); // Wait for both tasks to complete await Task.WhenAll(weatherTask, newsTask); // Display the results Console.WriteLine("Weather:"); Console.WriteLine(weatherTask.Result); Console.WriteLine(); Console.WriteLine("News:"); Console.WriteLine(newsTask.Result); Console.WriteLine("\nPress any key to exit..."); Console.ReadKey(); }
Now you should be getting the result of both API calls asynchronously as your output. This would look like this:
Weather: Weather Data: {"coord":{"lon":-0.1257,"lat":51.5085},"weather":[{"id":803,"main":"Clouds","description":"broken clouds","icon":"04d"}],"base":"stations","main":{"temp":284.69,"feels_like":283.81,"temp_min":283.57,"temp_max":285.67,"pressure":1022,"humidity":73},"visibility":10000,"wind":{"speed":7.2,"deg":280},"clouds":{"all":75},"dt":1675173675,"sys":{"type":2,"id":2075535,"country":"GB","sunrise":1675150859,"sunset":1675183598},"timezone":0,"id":2643743,"name":"London","cod":200} News: News Articles: {"status":"ok","totalResults":35,"articles":[{"source":{"id":"associated-press","name":"Associated Press"},"author":"Riaz Khan","title":"Pakistan blames 'security lapse' for mosque blast; 100 dead - The Associated Press - en Español","description":"PESHAWAR, Pakistan (AP) - A suicide bombing that struck inside a mosque at a police and government compound in northwest Pakistan reflects "security lapses," current and former officials said as the death toll from the devastating blast climbed to 100 on Tues.","url":"https://apnews.com/article/pakistan-mosque-bombing-death-toll-rises-fdbc592dfd3b9a23ec2081b635d65b6e","urlToImage":"https://storage.googleapis.com/afs-prod/media/9f4f3b1522cb4c39a45b6fa34d8d4f4c/3000.webp","publishedAt":"2023-01-31T12:08:29Z","content":"PESHAWAR, Pakistan (AP) A suicide bombing that struck inside a mosque at a police and government compound in northwest Pakistan reflects security lapses, current and former officials said as the deat. [+6786 chars]"},{"source":{"id":"reuters","name":"Reuters"},"author":null,"title":"Adani's $2.5 bln offer rides out share storm with investor backing - Reuters","description":"Gautam Adani's $2.5 billion share sale was <a href=\"/markets/deals/indias-adani-enterprises-enters-final-day-crucial-25-bln-share-sale-2023-01-31/\">fully subscribed</a> on Tuesday as investors pumped funds into his flagship Adani Enterprises Ltd <a href=\"http.","url":"https://www.reuters.com/markets/deals/indias-adani-enterprises-enters-final-day-crucial-25-bln-share-sale-2023-01-31/","urlToImage":"https://www.reuters.com/resizer/YBnDdAop85jNgVxJpQ3l4Dot4v4=/1200x628/smart/filters:quality(80)/cloudfront-us-east-2.images.arcpublishing.com/reuters/JBXYB5J3XJJGVK3J5W762M4I7U.jpg","publishedAt":"2023-01-31T10:35:00Z","content":"BENGALURU, Jan 31 (Reuters) - Gautam Adani's $2.5 billion share sale was fully subscribed on Tuesday as investors pumped funds into his flagship Adani Enterprises Ltd (ADEL.NS) despite a scathing rep. [+2224 chars]"},{"source":{"id":null,"name":"The Cincinnati Enquirer"},"author":"Dave Clark","title":"Watch: Frank Clark takes extra steps before fourth-quarter late hit of Joe Burrow - The Cincinnati Enquirer","description":"\"You see a little tough love right there from Frank Clark to his buddy Joe Burrow,\" CBS analyst Tony Romo said after Clark dropped Burrow.","url":"https://www.cincinnati.com/story/sports/nfl/bengals/2023/01/30/frank-clarks-fourth-quarter-late-hit-of-joe-burrow-not-called-by-afc-championship-officials/69853178007/","urlToImage":"https://www.gannett-cdn.com/presto/2023/01/30/PCIN/4f480ae2-3f9b-4fe0-87d2-70ccdc2c3572-012923BengalsChiefsAFCC_64.JPG?crop=4681,2634,x0,y237&width=3200&height=1801&format=pjpg&auto=webp","publishedAt":"2023-01-31T10:28:45Z","content":"With about nine minutes remaining in Sunday's AFC championship between Kansas City and Cincinnati, Chiefs defensive end Frank Clark appeared to take an extra three or four steps and push Bengals quar. [+516 chars]"},{"source":{"id":"cnn","name":"CNN"},"author":"Aya Elamroussi","title":"Millions across the South and central US brace for ice storm as officials urge staying off roads - CNN","description":"A winter storm bringing the triple threat of ice, sleet and snow Tuesday to parts of the South and central US has prompted officials to close roads and schools as they urge people to avoid traveling in dangerous conditions.","url":"https://www.cnn.com/2023/01/31/weather/winter-ice-storm-south-central-us/index.html","urlToImage":"https://media.cnn.com/api/v1/images/stellar/prod/230131064839-02-winter-weather-0130-tx.jpg?c=16x9&q=w_800,c_fill","publishedAt":"2023-01-31T09:38:00Z","content":"A winter storm bringing the triple threat of ice, sleet and snow Tuesday to parts of the South and central US has prompted officials to close roads and schools as they urge people to avoid traveling . [+4404 chars]"},{"source":{"id":"reuters","name":"Reuters"},"author":null,"title":"U.S. stops granting export licenses for China's Huawei - sources - Reuters","description":"The Biden administration has stopped approving licenses for U.S. companies to export most items to China's Huawei, according to three people familiar with the matter.","url":"https://www.reuters.com/technology/us-stops-provision-licences-export-chinas-huawei-ft-2023-01-30/","urlToImage":"https://www.reuters.com/resizer/mPA-jHfDSX5m9HZb7wtNU2yng-w=/1200x628/smart/filters:quality(80)/cloudfront-us-east-2.images.arcpublishing.com/reuters/2X5Z7LAB3VNQ7CHOQFOCVH274A.jpg","publishedAt":"2023-01-31T08:48:00Z","content":"Jan 30 (Reuters) - The Biden administration has stopped approving licenses for U.S. companies to export most items to China's Huawei, according to three people familiar with the matter.\r\nHuawei has f. [+2786 chars]"},{"source":{"id":null,"name":"CNBC"},"author":"Lee Ying Shan","title":"Asia-Pacific shares trade mostly lower; China manufacturing activity shows growth - CNBC","description":"Asia-Pacific shares trade mostly lower as China reports growth in manufacturing activity after three months of contraction","url":"https://www.cnbc.com/2023/01/31/asia-pacific-shares-fed-earnings-economic-data.html","urlToImage":"https://image.cnbcfm.com/api/v1/image/107179165-1673938740082-gettyimages-1245952422-SHANGHAI_ECONOMY.jpeg?v=1675122221&w=1920&h=1080","publishedAt":"2023-01-31T07:27:00Z","content":"Investors should fade the early 2023 rally, warned JPMorgan's top market strategist Marko Kolanovic.\r\nThe first quarter will likely mark a turning point for the market and its upward trajectory proba. [+1254 chars]"},{"source":{"id":"cnn","name":"CNN"},"author":"","title":"CNN goes to Ukraine front lines with key drone unit - CNN","description":"CNN's Fred Pleitgen embeds with Ukrainian drone operators on the front lines of Ukraine's war against Russia - a dangerous role that is crucial in detecting Russian forces' movements.","url":"https://www.cnn.com/videos/world/2023/01/30/ukraine-russia-drone-forest-pleitgen-pkg-sr-vpx.cnn","urlToImage":"https://media.cnn.com/api/v1/images/stellar/prod/230130175050-ukraine-pleitgen.jpg?c=16x9&q=w_800,c_fill","publishedAt":"2023-01-31T05:53:35Z","content":null},{"source":{"id":null,"name":"San Francisco Chronicle"},"author":"Matthias Gafni","title":"Wife's statement, crash video lead to charges for dad who drove Tesla off cliff with family inside - San Francisco Chronicle","description":"Prosecutors said they expect to file charges against Dharmesh Patel, the man accused of intentionally driving his Tesla off a cliff with his family inside.","url":"https://www.sfchronicle.com/crime/article/man-accused-of-intentionally-driving-tesla-off-a-17751626.php","urlToImage":"https://s.hdnux.com/photos/01/31/25/26/23418271/3/rawImage.jpg","publishedAt":"2023-01-31T05:33:33Z","content":"After visiting family in the Bay Area in early January, Dharmesh Patel drove southbound through the Tom Lantos Tunnel with his wife and two young kids.Suddenly, his white Tesla veered onto the dirt s. [+5718 chars]"},{"source":{"id":null,"name":"CNBC"},"author":"Amelia Lucas","title":"McDonald's is about to report earnings. Here's what to expect - CNBC","description":"McDonald's is set to report fourth-quarter earnings before the opening bell.","url":"https://www.cnbc.com/2023/01/31/mcdonalds-mcd-q4-2022-earnings.html","urlToImage":"https://image.cnbcfm.com/api/v1/image/107093385-1658759164183-gettyimages-1394272123-0j7a4791_f67c3191-2ced-493c-84a3-6c53a6a2fdbf.jpeg?v=1675141261&w=1920&h=1080","publishedAt":"2023-01-31T05:01:01Z","content":"McDonald's is expected to report its fourth-quarter earnings before the bell Tuesday.\r\nHere's what analysts surveyed by Refinitiv are expecting the company to report:\r\n<ul><li>Earnings per share: $2.. [+1069 chars]"},{"source":{"id":null,"name":"ESPN"},"author":"Tim MacMahon","title":"Luka Doncic drops 53 in Mavs' win while chirping with Pistons assistant - ESPN","description":"Luka Doncic posted his NBA-leading fourth 50-point game of the season to lead the Mavs to victory Monday night -- all while continuously chirping with Pistons assistant coach Jerome Allen.","url":"https://www.espn.com/nba/story/_/id/35561524/luka-doncic-drops-53-mavs-win-chirping-pistons-assistant","urlToImage":"https://a2.espncdn.com/combiner/i?img=%2Fphoto%2F2023%2F0131%2Fr1124723_1296x729_16%2D9.jpg","publishedAt":"2023-01-31T04:29:29Z","content":"DALLAS -- Luka Doncic made a detour toward the Detroit Pistons' bench during a timeout with 7.1 seconds remaining in the Dallas Mavericks' 111-105 win Monday night.\r\nHe approached Pistons assistant c. [+4922 chars]"},{"source":{"id":null,"name":"YourTango"},"author":"Ruby Miranda","title":"The 3 Zodiac Signs With Rough Horoscopes On January 31, 2023 - YourTango","description":"These three zodiac signs have rough horoscopes on January 31, 2023, and boredom is killing their joy.","url":"https://www.yourtango.com/2023358265/zodiac-signs-rough-horoscopes-january-31-2023","urlToImage":"https://www.yourtango.com/sites/default/files/styles/listing_big/public/image_blog/zodiac-signs-rough-horoscopes-january-31-2023.png?itok=96i5RUv-","publishedAt":"2023-01-31T04:02:50Z","content":"There's an interesting juxtaposition going on on January 31, 2023. We are working with the cosmic influence of Sun trine Moon, which is ordinarily a very good transit, but there's a downside to this . [+4460 chars]"},{"source":{"id":null,"name":"SciTechDaily"},"author":null,"title":"The Tragic Toll: COVID-19 Is a Leading Cause of Death in Children and Young People in the U.S. - SciTechDaily","description":"Between August 2021 and July 2022, COVID-19 was a leading cause of death in children and young people in the US, ranking eighth overall. COVID-19 was the top cause of death in children from an infectious disease, in the same period. Deaths in children from CO.","url":"https://scitechdaily.com/the-tragic-toll-covid-19-is-a-leading-cause-of-death-in-children-and-young-people-in-the-u-s/","urlToImage":"https://scitechdaily.com/images/COVID-19-Deaths-Timeseries.png","publishedAt":"2023-01-31T03:32:40Z","content":"ByUniversity of OxfordJanuary 30, 2023\r\nMonthly number of deaths in the US of children and young people (aged 0-19), where COVID-19 was listed as the cause of death on the death certificate. Credit: . [+9187 chars]"},{"source":{"id":"reuters","name":"Reuters"},"author":null,"title":"Western allies differ over jets for Ukraine as Russia claims gains - Reuters","description":"Ukraine's defence minister is expected in Paris on Tuesday to meet President Emmanuel Macron amid a debate among Kyiv's allies over whether to provide fighter jets for its war against Russia, after U.S. President Joe Biden ruled out giving F-16s.","url":"https://www.reuters.com/world/europe/biden-says-no-f-16s-ukraine-russia-claims-gains-2023-01-31/","urlToImage":"https://www.reuters.com/resizer/WcjjYRJmGzcnYZ4Hw7ACtIkWf_4=/1200x628/smart/filters:quality(80)/cloudfront-us-east-2.images.arcpublishing.com/reuters/OJVACH2ZAJOYBGRW6YORX2INO4.jpg","publishedAt":"2023-01-31T03:32:00Z","content":"KYIV, Jan 31 (Reuters) - Ukraine's defence minister is expected in Paris on Tuesday to meet President Emmanuel Macron amid a debate among Kyiv's allies over whether to provide fighter jets for its wa. [+5083 chars]"},{"source":{"id":"al-jazeera-english","name":"Al Jazeera English"},"author":"Al Jazeera","title":"`Surprisingly resilient': IMF lifts global growth forecasts - Al Jazeera English","description":"Fund says demand in the US and Europe has been stronger than expected, and that only the UK faces a recession.","url":"https://www.aljazeera.com/economy/2023/1/31/surprisingly-resilient-imf-lifts-global-growth-forecasts","urlToImage":"https://www.aljazeera.com/wp-content/uploads/2023/01/AP23030815831385.jpg?resize=1920%2C1440","publishedAt":"2023-01-31T02:44:43Z","content":"The International Monetary Fund (IMF) has raised its 2023 global growth outlook slightly due to surprisingly resilient demand in the United States and Europe and the reopening of Chinas economy after. [+4956 chars]"},{"source":{"id":null,"name":"YouTube"},"author":null,"title":"Two more Memphis officers relieved of duty after Tyre Nichols' death - CBS News","description":"Officials said Monday that two more Memphis officers were relieved of duty and three fire department employees were fired following the police beating of Tyr...","url":"https://www.youtube.com/watch?v=qdqkF_4KrH8","urlToImage":"https://i.ytimg.com/vi/qdqkF_4KrH8/maxresdefault.jpg","publishedAt":"2023-01-31T02:37:50Z","content":null},{"source":{"id":null,"name":"NBC Chicago"},"author":"Matt Stefanski","title":"Suburban School Worker Stole 11K Cases of Chicken Wings in $1.5M Embezzlement Scheme: Court Docs - NBC Chicago","description":"Prosecutors have charged the former head of food services at an Illinois school district of engaging in a massive embezzlement scheme.","url":"https://www.nbcchicago.com/news/local/suburban-school-cafeteria-worker-stole-11k-cases-of-chicken-wings-in-1-5m-embezzlement-scheme-prosecutors/3058487/","urlToImage":"https://media.nbcchicago.com/2023/01/chicken-wings-1.jpg?quality=85&strip=all&resize=1200%2C675","publishedAt":"2023-01-31T02:10:28Z","content":"Cook County prosecutors have charged the former head of food services at a south suburban school district of engaging in a massive embezzlement scheme in which she allegedly stole more than 11,000 ca. [+1893 chars]"},{"source":{"id":null,"name":"BBC News"},"author":"Isabelle Gerretsen","title":"Should you sing when suffering from a cold? - BBC","description":"Respiratory infections such as the common cold or flu can play havoc with our voices, but is it still safe to sing? And does Covid-19 represent a new threat?","url":"https://www.bbc.com/future/article/20230130-should-you-sing-when-suffering-from-a-cold","urlToImage":"https://ychef.files.bbci.co.uk/live/624x351/p0dzwy2z.jpg","publishedAt":"2023-01-31T01:04:52Z","content":"Vocal fold nodules are another concern for many singers, but they aren't caused by colds or infections, according to Costello.\r\n\"Nodules develop over time when a singer brings their vocal folds toget. [+2354 chars]"},{"source":{"id":null,"name":"Yahoo Entertainment"},"author":"Mario Parker and Zoe Tillman","title":"Trump Sues Journalist Bob Woodward for Releasing Interview Recordings - Yahoo News","description":"(Bloomberg) -- Former President Donald Trump is suing journalist Bob Woodward for releasing recordings of interviews that he gave to the journalist in 2019...","url":"https://news.yahoo.com/trump-sues-journalist-bob-woodward-210201503.html","urlToImage":"https://s.yimg.com/ny/api/res/1.2/VznkLmLJV.VTbNlI1ACmew--/YXBwaWQ9aGlnaGxhbmRlcjt3PTEyMDA7aD04MDA-/https://media.zenfs.com/en/bloomberg_politics_602/7b2cbb2bb0113d8e817a9e8615eaee09","publishedAt":"2023-01-31T00:09:00Z","content":"(Bloomberg) -- Former President Donald Trump is suing journalist Bob Woodward for releasing recordings of interviews that he gave to the journalist in 2019 and 2020, claiming he never agreed to those. [+4676 chars]"},{"source":{"id":null,"name":"YouTube"},"author":null,"title":"New Florida bill would allow concealed carry without permit - Action News Jax (CBS47 & FOX30)","description":"A new bill announced in the State Capitol Monday morning would allow for lawful gun owners to carry concealed firearms in public without a concealed carry pe...","url":"https://www.youtube.com/watch?v=DrC_VLR0Me4","urlToImage":"https://i.ytimg.com/vi/DrC_VLR0Me4/hqdefault.jpg","publishedAt":"2023-01-31T00:06:24Z","content":null},{"source":{"id":null,"name":"The Guardian"},"author":"Guardian staff reporter","title":"Russia-Ukraine war at a glance: what we know on day 342 of the invasion - The Guardian","description":"Russian attacks on Bakhmut and Donetsk; US will not provide F-16 fighters, says Biden","url":"https://www.theguardian.com/world/2023/jan/31/russia-ukraine-war-at-a-glance-what-we-know-on-day-342-of-the-invasion","urlToImage":"https://i.guim.co.uk/img/media/5703e666daeba2a05cd582df602915176c97a836/0_0_5000_3001/master/5000.jpg?width=1200&height=630&quality=85&auto=format&fit=crop&overlay-align=bottom%2Cleft&overlay-width=100p&overlay-base64=L2ltZy9zdGF0aWMvb3ZlcmxheXMvdGctZGVmYXVsdC5wbmc&enable=upscale&s=0c0c89bac9c0b88acf7e030e5f7adbfc","publishedAt":"2023-01-30T23:58:00Z","content":"<li>The United States will not provide the F-16 fighter jets that Ukraine has sought in its fight against Russia, President Joe Biden said on Monday, as Russian forces claimed a series of incremental. [+5630 chars]"}]}
static async Task<string> GetNewsAsync() { var result = string.Empty; var client = new HttpClient(); try { var newsTask = client.GetStringAsync("https://newsapi.org/v2/top-headlines?country=us&apiKey=YOURAPIKEY"); var newsResult = await newsTask; result = "News Articles: " + newsResult; } catch (Exception ex) { Console.WriteLine($"Error getting news articles: {ex.Message}"); } return result; }
If we have this error, the result of running the program again would be the following:
Error getting news articles: Response status code does not indicate success: 400 (Bad Request). Weather: Weather Data: {"coord":{"lon":-0.1257,"lat":51.5085},"weather":[{"id":803,"main":"Clouds","description":"broken clouds","icon":"04d"}],"base":"stations","main":{"temp":284.76,"feels_like":283.83,"temp_min":283.57,"temp_max":285.67,"pressure":1022,"humidity":71},"visibility":10000,"wind":{"speed":7.2,"deg":280},"clouds":{"all":75},"dt":1675174127,"sys":{"type":2,"id":2075535,"country":"GB","sunrise":1675150859,"sunset":1675183598},"timezone":0,"id":2643743,"name":"London","cod":200} News: Press any key to exit...
Generally, if that is your case, if you simply type into your search bar the URL from your GetStringAsync() method. So something like this: https://newsapi.org/v2/top-headlines?country=us&apiKey=YOURAPIKEY. (Make sure to change “YOURAPIKEY” for your actual API key) And then rerun the program; it will succeed in most cases.
Naturally, if that is still not the case, let us know in the comments below!
Great! However, this might look an awful lot like multithreading to you. And for sure, that is understandable, but let me explain why it is not the same.
Differences and similarities between multithreading and asynchronous programming.
Multithreading and asynchronous programming are often used interchangeably, but they are not exactly the same thing. Multithreading involves running multiple threads in parallel, while asynchronous programming involves executing tasks in a non-blocking way, allowing the program to continue executing other tasks while the asynchronous task is running in the background.
One similarity between multithreading and asynchronous programming is that they both allow you to run multiple tasks simultaneously, improving the performance and responsiveness of your applications.
In C#, asynchronous programming can be implemented using the async
and await
keywords. This makes it easier to write asynchronous code and provides a cleaner syntax compared to traditional multithreading.
If you want to learn more about multithreading in C#, check out our article on Multithreading in C#!
And that’s it! With these concepts in mind, you should now understand the difference and similarities between multithreading and asynchronous programming in C#.
Conclusion: Introduction to Asynchronous Programming with C#
Async programming is a powerful tool that allows developers to write efficient, responsive applications. By breaking down long-running tasks into smaller, asynchronous tasks, we can create quick and responsive applications, even when dealing with large amounts of data. In this article, we have seen how to use the async and await keywords to create asynchronous tasks and how to wait for them to complete. With this knowledge, you can now create your own asynchronous applications and improve the user experience of your applications.