Sending a C# Post Request: A Step-by-Step Guide
Sending a C# post request to an API is a common task when working with web services, and with C#, it’s easy to accomplish this task using the built-in JSON converter.
In this article, we will create a simple project demonstrating how to send a Post request to an API in C#, and how to serialize and deserialize C# objects to and from JSON using the System.Text.Json namespace.
Creating a New Console Application in Visual Studio
First, we will start by creating a new console application in Visual Studio. We will name it “PostApiDemo”. This step is necessary to create the foundation for our project and have a place to add our code.
- Open Visual Studio and click on “Create a new project.”
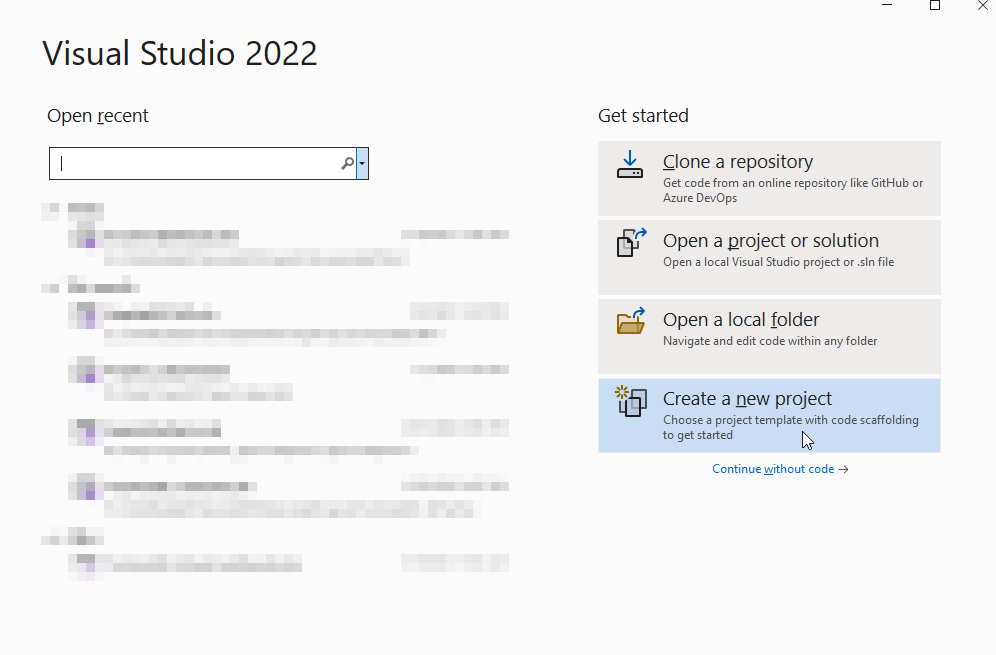
- Select “Console App (.NET 6)” from the list of templates.
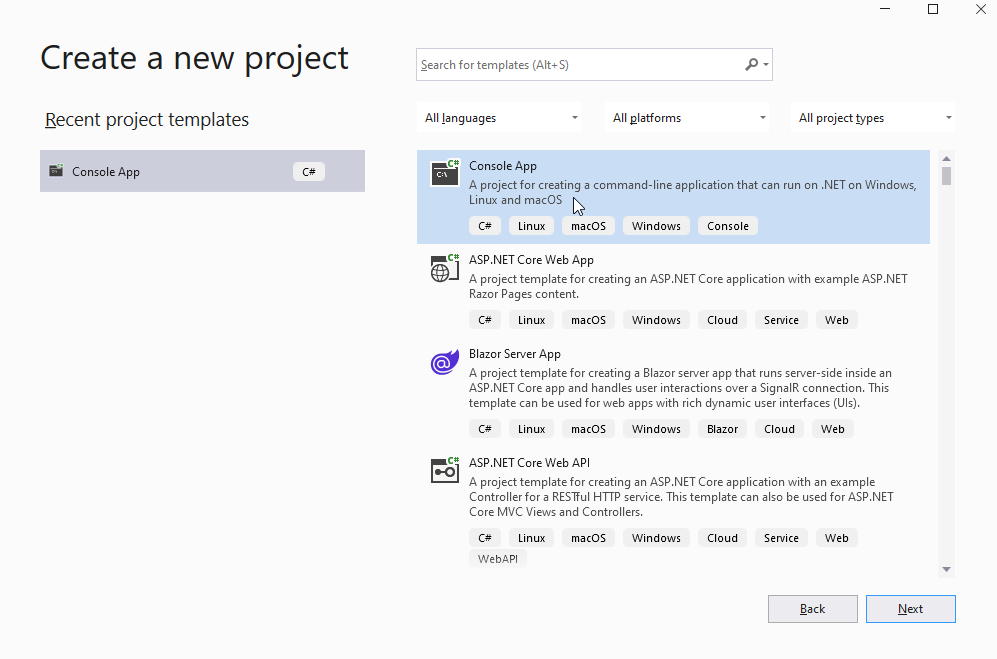
- Give your project a name, for example “PostApiDemo” and click on “Create.”
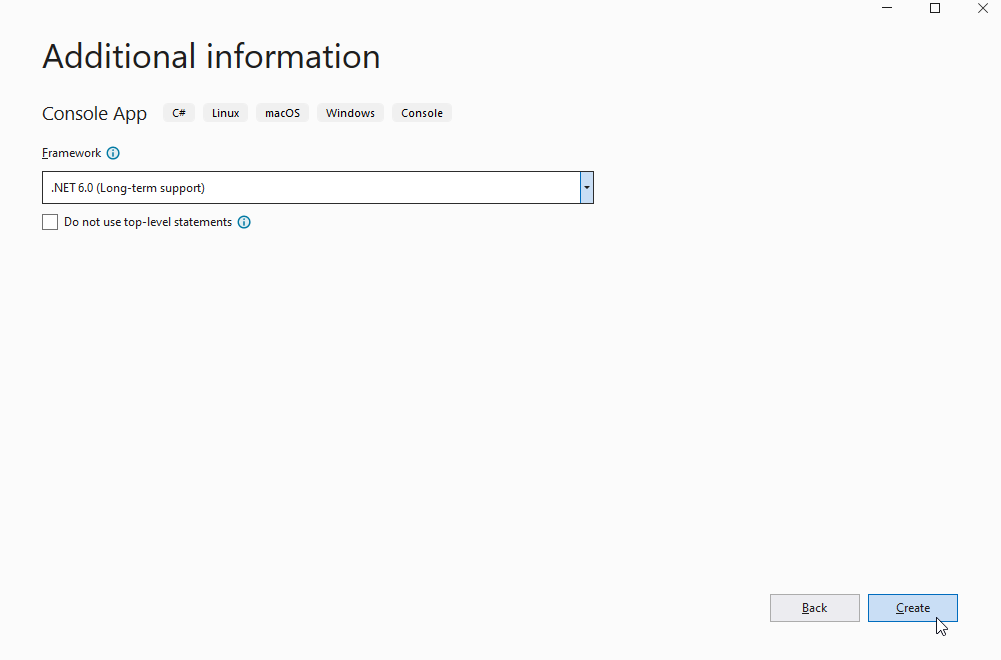
Defining the Structure of the Data to be sent to the API
Next, we will create a class called “PostData” that will represent the data we want to send to the API as a JSON object. This class will have properties such as “Name”, “Age”, and “Address”. This step is necessary to define the structure of the data that we will be sending to the API.
class PostData { public string Name { get; set; } public int Age { get; set; } public string Address { get; set; } }
We will also create another class called “PostResponse” that will represent the response we get from the API. This class will have a property “Id” which will hold the Id of the newly created resource. This step is necessary to define the structure of the data we will receive from the API.
class PostResponse { public int Id { get; set; } }
Creating an HttpClient Object and Setting the API Base Address
Now we can move on to our Main method, where we will create an instance of the PostData class we just created. This step is necessary to create a new class instance and populate it with the data we want to send to the API.
var postData = new PostData { Name = "John Doe", Age = 30, Address = "123 Main St" };
Next, we will create a new HttpClient object, allowing us to make the POST request. We will set the base address of the API and then use the System.Text.Json namespace to serialize our postData object to a JSON string. This step is necessary to create the HttpClient object that will handle the connection to the API and to convert the C# object to a JSON string that can be sent in the request.
We are POSTING to https://jsonplaceholder.typicode.com/, a free fake API for testing and prototyping. Evidently, if you need to post to a different URI, you can change this address to whichever you need.
var client = new HttpClient(); client.BaseAddress = new Uri("https://jsonplaceholder.typicode.com/"); var json = System.Text.Json.JsonSerializer.Serialize(postData); var content = new StringContent(json, Encoding.UTF8, "application/json");
How to send a C# post request
Next, we will make the actual POST request by calling the HttpClient’s “PostAsync” method. This method takes the API’s endpoint and the HttpContent object as its parameters. This step is necessary to send the request to the API with the data we want to post.
var response = client.PostAsync("posts", content).Result;
Checking the Response and Deserializing the JSON Response
Once we have the response, we can check the status code to see if the request was successful. If it was, we can read the response content and use the System.Text.Json namespace to deserialize it back to a C# object. This step is necessary to check if the request was successful and convert the JSON response back to a C# object that we can work with.
if (response.IsSuccessStatusCode) { var responseContent = response.Content.ReadAsStringAsync().Result; var options = new JsonSerializerOptions { PropertyNameCaseInsensitive = true }; var postResponse = System.Text.Json.JsonSerializer.Deserialize<PostResponse>(responseContent, options); Console.WriteLine("Post successful! ID: " + postResponse.Id); } else { Console.WriteLine("Error: " + response.StatusCode); }
If we now start the application we should see the “Id” of our created record.
Conclusion: Sending a C# Post Request
That’s it for this article. By now you should have a decent knowledge of how to send a C# post request to an API.
If you’re new to C# and collections, it’s always a good idea to start with basic examples and then build on them as you gain more experience. And don’t forget that when in doubt, you can always refer to this article to help you understand How to call Post API in C#. Also, if you are interested in more API related topics, check out this article about calling GET API in C#!
Oh, and if you feel overwhelmed with coding, check out our developer membership (seriously, it’s worth it!). We help you master coding fast and easily.